Learn C++ Programming Language
Polymorphism and Virtual Members Functions
Definition of polymorphism and virtual members functions
Virtual functions allow the most specific version of a member function in an inheritance hierarchy to be selected for execution. Virtual functions make polymorphism possible.
A piece of code is said to be polymorphic if executing the code with different types of data produces different behavior.
For example, a function would be called polymorphic if it executes differently when it is passed different types of parameters.
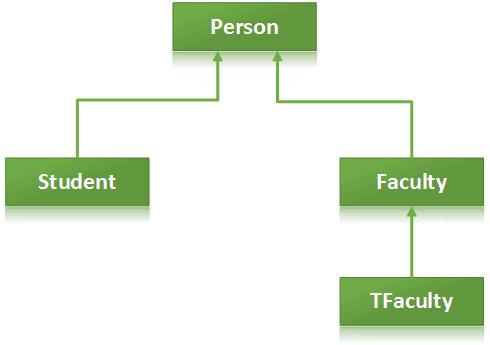
The following program is a program where the getName function of the Person class has been declared virtual. It includes the inheritance5.h file, which make the getName function in the Person class virtual.
Contents of Inheritance5.h
#include <iostream>
#include <string>
using namespace std;
enum Discipline { ARCHEOLOGY, BIOLOGY, COMPUTER_SCIENCE };
enum Classification { FRESHMAN, SOPHOMORE, JUNIOR, SENIOR };
class Person{
protected:
string name;
public:
Person() { setName(""); }
Person(string pName) { setName(pName); }
void setName(string pName) { name = pName; }
// Virtual function.
virtual string getName() { return name; }
};
class Student:public Person {
private:
Discipline major;
Person *advisor;
public:
Student(string sname, Discipline d, Person *adv)
: Person(sname) {
major = d;
advisor = adv;
}
void setMajor(Discipline d) { major = d; }
Discipline getMajor() { return major; }
void setAdvisor(Person *p) { advisor = p; }
Person *getAdvisor() { return advisor; }
};
class Faculty:public Person {
private:
Discipline department;
public:
Faculty(string fname, Discipline d) : Person(fname) {
department = d;
}
void setDepartment(Discipline d) { department = d; }
Discipline getDepartment( ) { return department; }
};
class TFaculty : public Faculty {
private:
string title;
public:
TFaculty(string fname, Discipline d, string title)
: Faculty(fname, d){
setTitle(title);
}
void setTitle(string title) { this->title = title; }
// Virtual function
virtual string getName( ) {
return title + " " + Person::getName();
}
};
This program demonstrates the polymorphic behaviour of classes with virtual functions.
#include <iostream>
#include "inheritance5.h"
using namespace std;
int main() {
// Create an array of pointers to Person objects
const int NUM_PEOPLE = 5;
Person *arr[NUM_PEOPLE] = {
new TFaculty("Indiana Jones", ARCHEOLOGY, "Dr."),
new Student("Tom Cruise", COMPUTER_SCIENCE, NULL),
new Faculty("James Coukc", BIOLOGY),
new TFaculty("Sharon Stone", BIOLOGY, "Professor"),
new TFaculty("Nicole Kidman", ARCHEOLOGY, "Dr.")
};
// Print the names of the Person objects
for (int k = 0; k < NUM_PEOPLE; k++) {
cout << arr[k]->getName() << endl;
}
return 0;
}
Program Output :
Indiana Jones
Tom Cruise
James Couck
Sharon Stone
Nicole Kidman
The virtual characteristic is inherited: that is, if a member function of a derived class overrides a virtual function in the base class, then that member function is automatically virtual itself.
Although it is not necessary, many programmers tag all virtual functions with the key word virtual to make it easer to identify them.
Ads Right