Learn C++ Programming Language
Inheritance in C++ programming
Definition of inheritance
When one object is a specialized version of another object, there is an is-a relationship between them. For example, a grasshopper is an insect. Here are a few other examples of the is-a relationship.
- A poodle is a dog.
- A car is a vehicle.
- A rectangle is a shape.
When an is-a relationship exists between objects, it means that the specialized object has all of the characteristics of the general object, plus additional characteristics that make it special.
In object-oriented programming, inheritance is used to create an is-a relationship between classes.
Inheritance involves a base class and a derived class . The base class is the general class and the derived class is the specialized class.
The derived class is based on, or derived from, the base class. You can think of the base class as the parent and the derived class as the child.
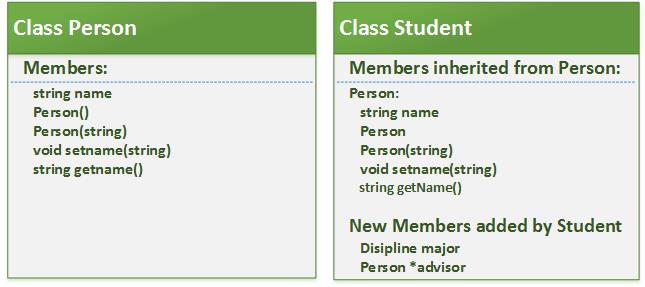
The inheritance is illustrated in this figure in the case of the Student and Person classes. The following program demonstrates the creation and use of an object of a derived class by creating a Faculty object.
The program uses arrays of strings that map values of enumerated types to strings to enable printing of values of enumerated types in the form of strings.
The included “inheritance.h” file contains declarations of the Person, Student, and Faculty classes, as well as the enumerated types Discipline and Classification.
Contents of Inheritance.h
#include <iostream>
#include <string>
using namespace std;
enum Discipline { ARCHEOLOGY, BIOLOGY, COMPUTER_SCIENCE };
enum Classification { FRESHMAN, SOPHOMORE, JUNIOR, SENIOR };
class Person {
private:
string name;
public:
Person() { setName("");
}
Person(string pName) { setName(pName);
}
void setName(string pName) { name = pName; }
string getName() { return name; }
};
class Student:public Person{
private:
Discipline major;
Person *advisor;
public:
void setMajor(Discipline d) { major = d; }
Discipline getMajor() { return major; }
void setAdvisor(Person *p) { advisor = p; }
Person *getAdvisor() { return advisor; }
};
class Faculty:public Person {
private:
Discipline department;
public:
void setDepartment(Discipline d) { department = d; }
Discipline getDepartment( ) { return department; }
};
Objects and derived classes
This program demonstrates the creation and use of objects of derived classes.
#include <iostream>
#include "inheritance.h"
using namespace std;
const string dName[] = {
"Archeology", "Biology", "Computer Science"
};
const string cName[] = {
"Freshman", "Sophomore", "Junior", "Senior"
};
int main() {
// Create a Faculty object
Faculty prof;
// Use a Person member function
prof.setName("Indiana Jones");
// Use a Faculty member function
prof.setDepartment(ARCHEOLOGY);
cout << "Professor " << prof.getName()
<< " teaches in the " << "Department of ";
// Get department as an enumerated type
Discipline dept = prof.getDepartment();
// Print out the department in string form
cout << dName[dept] << endl;
return 0;
}
Program Output :
Professor Indiana Jones teaches in the Department of Archeology
The derived class inherits the member variables and member functions of the base class without any of them being rewritten.
Furthermore, new member variables and functions may be added to the derived class to make it more specialized than the base class.
Ads Right